使用node.js的sharp库写一个图片压缩工具
sharp库能将常见的大尺寸图像格式转换为尺寸更小、适合网络使用的 JPEG、PNG、WebP、GIF 和 AVIF 图像,这些图像的尺寸可以各不相同。
它可以在所有支持 Node-API v9 的 JavaScript 运行时环境中使用,包括 Node.js(^18.17.0 或 >= 20.3.0)、Deno 和 Bun。
由于使用了 libvips,图像缩放速度通常比采用最快参数的 ImageMagick 或 GraphicsMagick 还要快 4 到 5 倍。
色彩空间、嵌入的 ICC 配置文件和 alpha 透明通道都能得到正确处理。Lanczos 重采样确保在追求速度的同时不会牺牲质量。
除了图像缩放,还提供旋转、提取、合成和伽马校正等操作。
大多数现代 macOS、Windows 和 Linux 系统不需要任何额外的安装或运行时依赖项。
下面是一段用豆包生成的压缩图片的工具
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| const sharp = require('sharp'); const fs = require('fs'); const path = require('path');
function compressImage(inputPath, outputPath, quality = 80) { const ext = path.extname(inputPath).toLowerCase(); let image;
switch (ext) { case '.jpeg': case '.jpg': image = sharp(inputPath).jpeg({ quality }); break; case '.png': image = sharp(inputPath).png({ quality: quality }); break; case '.webp': image = sharp(inputPath).webp({ quality }); break; default: console.error('不支持的图片格式,请使用 JPEG、PNG 或 WebP 格式的图片。'); return; }
return image .toFile(outputPath) .then(() => { console.log(`图片压缩成功,保存到 ${outputPath}`); }) .catch((err) => { console.error('图片压缩失败:', err); }); }
const args = process.argv.slice(2); if (args.length < 2) { console.error('请提供输入图片路径和输出图片路径作为命令行参数。'); process.exit(1); }
const inputImagePath = args[0]; const outputImagePath = args[1]; const quality = parseInt(args[2], 10) || 80;
if (fs.existsSync(inputImagePath)) { compressImage(inputImagePath, outputImagePath, quality); } else { console.error('输入文件不存在,请检查路径。'); }
|
要使用这段代码,首先要安装node.js,安装最新的LTS版本就可以了。
然后创建一个sharp-demo 文件夹
之后进入sharp-demo文件夹,在终端输入npm init -y
初始化一个 package.json 文件。
修改package.json,添加一行启动命令"start": "node index.js"
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| { "name": "sharp-demo", "version": "1.0.0", "description": "", "license": "ISC", "author": "hejiangda", "type": "commonjs", "main": "index.js", "scripts": { "start": "node index.js" }, "dependencies": { "sharp": "^0.33.5" } }
|
接着创建一个index.js
文件,把代码填进去
最后在终端中运行:
1
| npm start input.png output.png
|
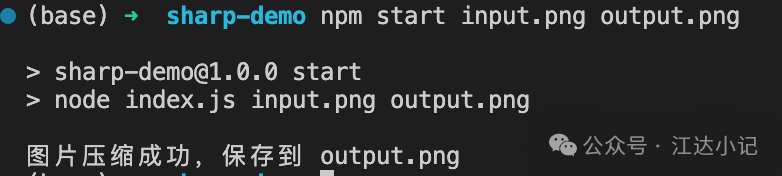
原图有1.1MB,压缩后是320KB:
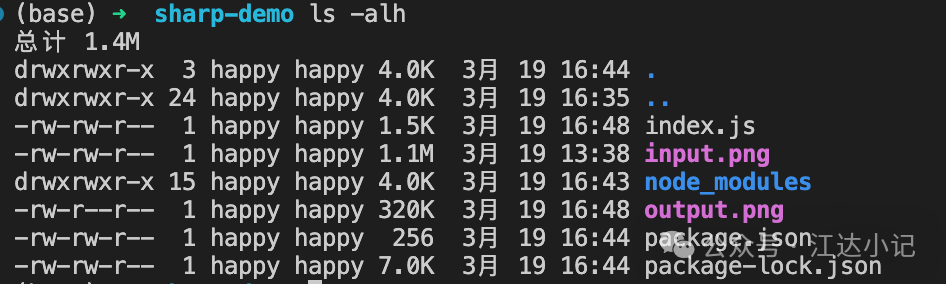
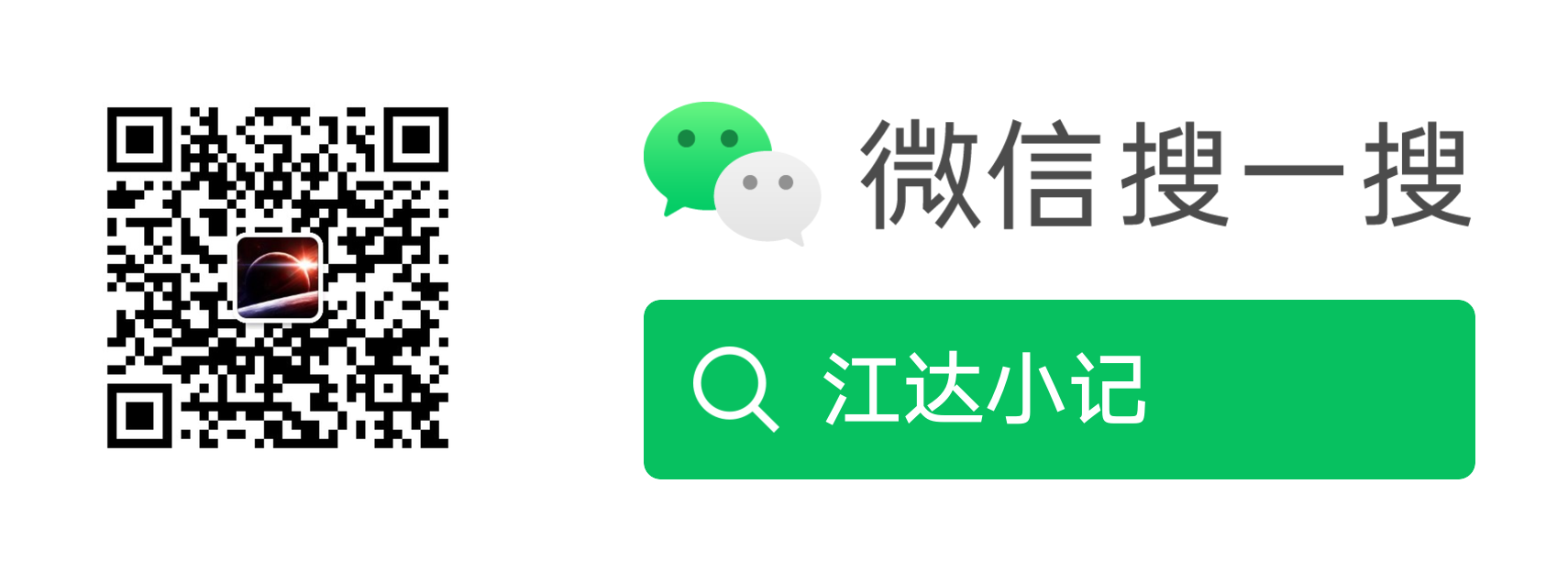